Category |
Difficulty |
Likes |
Dislikes |
algorithms |
Medium (66.80%) |
666 |
- |
Tags
Companies
给你二叉搜索树的根节点 root
,同时给定最小边界low
和最大边界 high
。通过修剪二叉搜索树,使得所有节点的值在[low, high]
中。修剪树 不应该 改变保留在树中的元素的相对结构 (即,如果没有被移除,原有的父代子代关系都应当保留)。 可以证明,存在 唯一的答案 。
所以结果应当返回修剪好的二叉搜索树的新的根节点。注意,根节点可能会根据给定的边界发生改变。
示例 1:

1 2
| 输入:root = [1,0,2], low = 1, high = 2 输出:[1,null,2]
|
示例 2:
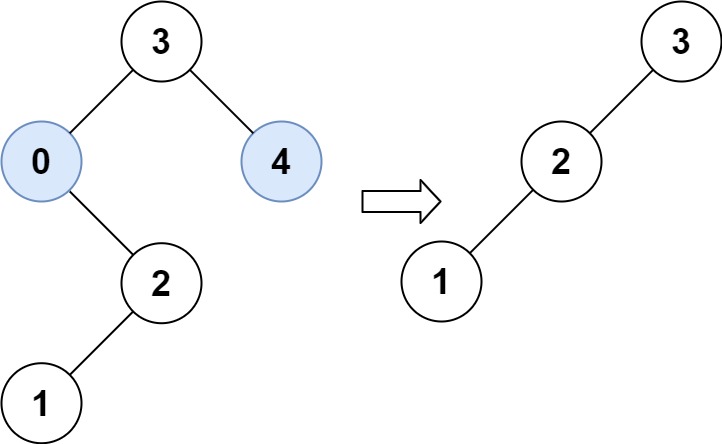
1 2
| 输入:root = [3,0,4,null,2,null,null,1], low = 1, high = 3 输出:[3,2,null,1]
|
提示:
- 树中节点数在范围
[1, 104]
内
0 <= Node.val <= 104
- 树中每个节点的值都是 唯一 的
- 题目数据保证输入是一棵有效的二叉搜索树
0 <= low <= high <= 104
模拟(c++)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86
| // @before-stub-for-debug-begin #include <vector> #include <string> #include "commoncppproblem669.h"
using namespace std; // @before-stub-for-debug-end
/* * @lc app=leetcode.cn id=669 lang=cpp * * [669] 修剪二叉搜索树 */
// @lc code=start /** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode() : val(0), left(nullptr), right(nullptr) {} * TreeNode(int x) : val(x), left(nullptr), right(nullptr) {} * TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {} * }; */
/** * 不在区间内的值被移除 * 因为是二叉搜索树 * root->val如果大于high,while(root->val>high)root=root->left * root->val <= high >= low, root->left = pre, pre = root->left, while(pre->val < low) pre = pre->right, tmp = pre, while() */ class Solution { public: void dfs(TreeNode *&root, int low, int high) { if (!root) { return ; } TreeNode *tmp = root->left; while (tmp && tmp->val < low) { if (tmp->right && (tmp->right->val >= low && tmp->right->val <= high)) { tmp = tmp->right; break; } else if (tmp->left && (tmp->left->val >= low && tmp->left->val <= high)) { tmp = tmp->left; break; } tmp = tmp->right; } root->left = tmp; tmp = root->right; while (tmp && tmp->val > high) { if (tmp->left && (tmp->left->val >= low && tmp->left->val <= high)) { tmp = tmp->left; break; } else if (tmp->right && (tmp->right->val >= low && tmp->right->val <= high)) { tmp = tmp->right; break; } tmp = tmp->left; } root->right = tmp; dfs(root->left, low, high); dfs(root->right, low, high); }
TreeNode* getRoot(TreeNode* root, int low, int high) { if (!root || (root->val >= low && root->val <= high)) { return root; } if (root->val > high) { return getRoot(root->left, low, high); } else { return getRoot(root->right, low, high); } }
TreeNode* trimBST(TreeNode* root, int low, int high) { if (!root) return nullptr; root = getRoot(root, low, high); dfs(root, low, high); return root; } }; // @lc code=end
|